Yahoo Answers is shutting down on May 4th, 2021 (Eastern Time) and beginning April 20th, 2021 (Eastern Time) the Yahoo Answers website will be in read-only mode. There will be no changes to other Yahoo properties or services, or your Yahoo account. You can find more information about the Yahoo Answers shutdown and how to download your data on this help page.
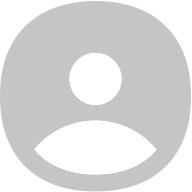
Craige
I am an aspiring web designer and blogger
What's the error in this piece of code. Here I'm trying to enter integers into an array using scanner class and output them.?
int[] salaries = new int[10];
Scanner scanner = new Scanner(System.in);
System.out.println("Enter 10 salaries");
for (int i = 0; i < salaries.length; i++) {
salaries[i] = scanner.nextInt();
}
for (int b : salaries) {
System.out.println(b);
}
}
1 AnswerProgramming & Design7 years agoJava program Using a hash mark and an end-of-line, write a program that produces the following shape:?
########
######
####
##
My code:
public static void main(String[] args) {
String space = " ";
int count = 8; // counter to track number of hashes
for (int i = 0; i < 4; i++) {
System.out.println();
for (int hash = 0; hash < count; hash++)
System.out.print("#");
count -= 2;
System.out.printf("%3s",space);
}
}
}
need help with adding the space on the new line so that the shape is aligned properly.
Thanks in advance.
2 AnswersProgramming & Design7 years agoJava classes question?
Write the code to print the income of all objects in the a array, where a contains objects of type Employee that have the getSalary method and objects of type Student that have the getStudentLoan method. Employees and students inherit from the Person class. Use the instanceof keyword to determine the type of the objects in the array.
Thanks in advance.
1 AnswerProgramming & Design7 years agoJava toString polymorphism question ?
Consider the following code.
Person p = new Person ( . . . . ) ;
Object o = p;
System . out . println (o . toString () ) ;
What will be printed if the Person class has the appropriate toString method? What will be printed if the Person class does not have the appropriate toString method? If the Person class has a toString method, then which toString method will be
executed, the one for the Person class or the one for the Object class? Explain why.
Can someone give me some help here
My responses:
the name of the person will be printed
nothing will be printed if person class doesn't have appropriate method
the Object method will be printed
since it is where the toString method prints to by default. Also output is o.toString and o = p would simply print the object and not take the Person class into consideration.
Thanks in advance.
1 AnswerProgramming & Design7 years agoWhy can't a for each loop be used to bubble sort in Java?
I'm writing a Bubble sort program in java but want to use a for each loop
My code:
public class BubbleSort {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
int size = 5;
for (int a = 1; a < size - 1;a++ ){
bubbleSort();
}
}
public static void bubbleSort(){
int [] a = {5,2,4,2,9};
for (int b : a ) {
if(a[1] < a[0]){
a[1] = a[0];
a[0] = a[1];
}
System.out.println(b);
}
}
}
I'm aware it can be written with two for loops to iterate through an array and an If construct to compare and swap consecutive elements until the array is in the right order.
Thanks
2 AnswersProgramming & Design7 years agoDifference between sports section in a newspaper and writing in a sports magazine?
I'm about to submit my column for a new newspaper and I'd like to understand thoroughly how they are different.
Thanks
2 AnswersMedia & Journalism7 years agoSecond largest double in ArrayList java?
Question:
Write a method that takes in an ArrayList of doubles. The method should return the second largest double in the ArrayList. You can assume that the ArrayList contains at least two elements. The signature of the method should be as follows:
public static double secondLargest ( ArrayList<Double> a)
My Code:
package pkgdouble.arraylist;
import java.util.ArrayList;
/**
*
* @author HP COMPAQ
*/
public class DoubleArraylist {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
ArrayList black = new ArrayList<>();
System.out.println(secondLargest(black));
}
public static double secondLargest(ArrayList<Double> a) {
double b = 0.0;
a.add(3, new Double(34.5));
a.add(4, new Double(14.5));
a.add(7, new Double(100.3));
return b;
}
}
I'm getting an index out of bounds exception error. Can someone help me understand it help with the solution as well.
Thanks
1 AnswerProgramming & Design7 years agoResult of two bytes multiplied and converted to an int in java?
some pseudocode here:
byte x = 13
byte y = 21
z = x*y
output z.
In java why would the answer be 24? I don't seem to understand why it's not 17 (since an int is 4 bytes) and z =( (13/4) * (21/4)).
Thanks
3 AnswersProgramming & Design7 years agoComplex numbers java?
Can someone help me with this complex numbers program:
class Complexn {
private double realPart, imaginaryPart;
public Complexn(double real, double imaginary) {
imaginary = this.imaginaryPart;
real = this.realPart;
}
public double addreal(Complexn num) {
double sum = 0;
return sum;
}
public double addimag(Complexn num) {
double sum = 0;
return sum;
}
public double subtractreal(Complexn num) {
double diff = 0;
return diff;
}
public double subtractimag(Complexn num) {
double diff = 0;
return diff;
}
public static double add(Complexn n1, Complexn n2) {
double sum = 0;
return sum;
}
private static double subtract(Complexn n1, Complexn n2) {
double diff = 0;
return diff;
}
@Override
public String toString() {
return this.realPart + "";
}
public boolean equality1(Complexn n1, Complexn n2) {
while (n1 == n2) {
return true;
}
return false;
}
}
class Main {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
Complexn n1 = new Complexn(2, 4);
Complexn n2 = new Complexn(22, 6);
double d1 = n1.addreal(n2);
double d2 = n2.subtractreal(n1);
double d4 = n1.addimag(n2);
double d5 = n2.subtractimag(n1);
3 AnswersProgramming & Design7 years agoProgrammer physique?
if someone is trying to achieve a high level of competency with their programming skills, is maintaining a good physique a realistic aim?
I am a bodybuilder and want to become a computer programmer as well. I'm worried that as the hours I put in coding increase ( or even on the average day for that matter), whether i,ll make any real increase in muscle mass and size.
2 AnswersTechnology7 years agoProgramming paradigms?
What are the limitations of each of the programming paradigms ( object-oriented, functional, procedural,etc) and give me some areas where different paradigms would be best suited.
I'm trying to gain some perspective on which programming languages are best for which tasks.
2 AnswersProgramming & Design7 years agoComplex number class in Java?
Write a class that is called Complex and that manipulates complex numbers.
• The class should have the variables realPart and imaginaryPart, which should be both of type double.
• The class should support the add method. For example, if c1 and c2 are objects of type Complex, then c1.add(c2) will return a Complex object that is the sum of the two objects. The constructed object will have a real part that is the sum of the real parts of the two objects, and an imaginary part that is the sum of the imaginary parts of the two objects.
• Similarly, write a subtract method. The constructed object will have a real part that is the difference of the real parts of the two objects, and an imaginary part that is the difference of the imaginary parts of the two objects.
• Write static versions of the add and subtract methods. For example, Complex.add(c1,c2) will return the result of adding two complex numbers. Note that now the hidden parameter is gone. Similarly, Complex.subtract(c1,c2) will return the difference of the two numbers.
• Write an empty constructor and a constructor that takes in the real and imaginary part of the number.
• Write a toString method that returns the complex number as a String. For example, it will return 3+2i when the real part is 3 and the imaginary part is 2.
• Add an equals method that compares two objects of type Complex for equality.
Create a second class with a main method and use it to test the methods of the Complex class.
Some help please
1 AnswerProgramming & Design7 years agoTwo dimensional square array in java?
Question:
Write a method that takes as input a two-dimensional square array of integers. The method should return the sum of the elements of the top-right to bottom-left diagonal. Write a full program that tests the method.
My code:
package two.dimensional.square.array;
public class TwoDimensionalSquareArray {
static int [][] numbers= { {2,4} , {5,6} };
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
System.out.println(squareArray (numbers));
}
public static int squareArray(int[][] a) {
int sum = 0;
for (int num[] : a) {//iterate through rows
for (int col : num) {//iterate through columns
sum = a[1][2] + a[2][1];
}
}
return sum;
}
}
The program compiles but doesn't work as I want it to. Can someone please help me understand why and suggest a solution to my problem?
Thanks
1 AnswerProgramming & Design7 years agoAverage of variable integers in Java?
Write a method that takes as input a variable number of integers. The method should return the average of the integers as a double. Write a full program to test the method.
My code:
package average.pkgint;
import java.util.Scanner;
public class AverageInt {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
Scanner b = new Scanner(System.in);
System.out.println("Enter your numbers:");
int number= b.nextInt();
System.out.println("The average is:"+Avnt());
}
public static int Avnt(int ... a){
int result = 1;
int sum = 0;
for (int num : a) {
sum += a[num];
int average = ( sum / num);
result =average;
}
return result;
}
}
Can someone help me with this. Arrays in methods have been a problem for me ( and using a variable number of arguments as well).
Thanks in advance.
1 AnswerProgramming & Design7 years agoJava,Number of occurrences of an int in an array?
Problem:
Write a method that finds the number of occurrences of the integer specified as the first parameter in the array of integers that is specified as the second parameter. Write a full program to test the method.
My code:
package number.of.integers;
import java.util.Scanner;
public class NumberOfIntegers {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
Scanner n = new Scanner(System.in);
// System.out.println("Now enter your code of integers for someone to find ");
int[] y = {2, 4, 6, 4, 26, 64, 252};
System.out.println("Enter a number");
int Num = n.nextInt();
while (true) {
System.out.println(NumOcc(Num, y));
}
}
public static int NumOcc(int number, int[] z) {
int a = 0;
if (number == z.length) {
System.out.println("Found!");
a++;
}
return a;
}
}
Can someone help me out with this program..I keep on getting zeros outputted and the compiler doesn't stop printing.
Thanks
2 AnswersProgramming & Design7 years agoWhat would be the correct code to output the following method? Java?
public static void stringcompose(String []s){
String r = "Hello there";
char convert [] = r.toCharArray();
System.out.println(convert);
}
Actually, the question here is:
Write a method that takes as input a string and returns the array of characters that compose the string. Write a full program to test the method. The expression s.charAt(i) returns the character at position i of the string s.
My code:
package stringto.pkgchar;
import java.lang.*;
public class StringtoChar {
static String [] v = new String [5];
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
System.out.println("Enter your word:");
for(int i=0,j=5;i<j;i++){
System.out.println(stringcompose());
}
}
public static void stringcompose(String []s){
String r = "Hello there";
char convert [] = r.toCharArray();
System.out.println(convert);
}
}
1 AnswerProgramming & Design7 years agoProgramming and java strings ( finding the largest in an array of strings?
I've got a question here. I've written the program however I'm getting an java.lang.ArrayIndexOutOfBoundsException: 10 error.
Question:
Write a method that takes as input an array of strings and returns the longest string.
Write a full program to test the method. Remember that s.length() will return the
length of the string s.
Program:
package largest.string;
import java.util.Scanner;
/**
*
* @author HP COMPAQ
*/
public class LargestString {
static String[] a = new String[10];
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
System.out.print("Enter 10 strings");
Length(a) ;
}
// System.out.println(a[i]);
public static String Length(String[] a) {
Scanner in = new Scanner(System.in);
int i;
int largest = 0;
for (i = 0; i < 10; i++) {
a[i] = in.next(); //looping through array
if (a[i].length() > largest) {//finding largest
largest = a[i].length();
}
}
return a[i];
}
}
Any help would be appreciated
2 AnswersProgramming & Design7 years agoBad operand types for binary integer question : java?
I've got a program here where I'm getting : Bad operand types for binary integer > error when I try to determine the longest string in a array of strings.
The program is as follows:
package largest.string;
import java.util.Scanner;
import java.util.Arrays;
/**
*
* @author HP COMPAQ
*/
public class LargestString {
static String[] a = new String[10];
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
// System.out.println(a[i]);
}
public static String Length(String[] a) {
Scanner in = new Scanner(System.in);
int i;
for ( i = 0; i < 10; i++) {
a[i] = in.next();
}
if (a > a.length) {
System.out.println(a[i]);
}
return a[i];
}
Would appreciate if someone cleared this for me.
1 AnswerProgramming & Design7 years agoJAVA : third smallest number in an array?
I need some help with this program.
Problem: Write a method that takes as input an array of integers and returns the third smallest number. Write a full program to test the method.
I need help fixing the out of bounds error my compiler reports.
My code:
package third.smallest.number;
/**
*
* @author HP COMPAQ
*/
public class ThirdSmallestNumber {
static final int MAX=10;
static int[] k = new int [MAX];
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
System.out.println("Enter 10 numbers");
for( int i =0,j=2; i<MAX;j++){
i = k[j];
}
System.out.println("The third smaller number is:" + thirdsmallest(k));
}
public static int thirdsmallest(int[] k){
int small= 0;
for(int pos:k){
small += pos;
}
return small;
}
}
Thanks
2 AnswersProgramming & Design7 years agomethod variable type in java?
IN java Platform SE 7, can the Method modifier and description be varied. In other words, take this example:
random() has a modifier of static double. however, i've seen program where it is used on int ( with a typecast ).
Here:
public static int randomnumber (int z){
int y = 1;
if (z>=0 && z<10){
y = (int) (Math.random() *10);
}
else {
System.out.println("Not in range");
}
return y;
}
it works on static int. Does that apply to other methods as well. Or does it only work with a typecast.
Thanks
2 AnswersProgramming & Design7 years ago