Yahoo Answers is shutting down on May 4th, 2021 (Eastern Time) and beginning April 20th, 2021 (Eastern Time) the Yahoo Answers website will be in read-only mode. There will be no changes to other Yahoo properties or services, or your Yahoo account. You can find more information about the Yahoo Answers shutdown and how to download your data on this help page.
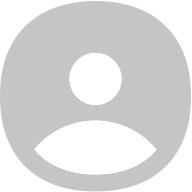
We have just started
Need help with programming in C?
I have notes that show a simple way to print out integer binary trees in this form
20(10(5()())(17()()))(30(21()())(*))
I want to write a program that reads in a binary tree in the above form and displays it in a ‘friendlier’ style
20----30
| |
10-17 21
|
5
The tree has left branches vertically down the page and right branches horizontally right
The binary tree is read in using argv[1](this is the string itself, NOT a filename), for example :% treevis "20()()"
I'm new to C and completely new to binary trees. Could you break down what I have to do for this project and explain. A push in the right direction would be greatly appreciated
1 AnswerProgramming & Design7 years agoReading from argv[1] in C?
What does that mean and how is it different from writing something in C normally. Could you demonstrate using simple examples. Thanks!
2 AnswersProgramming & Design7 years agoThe syntax of linked list in C programming?
I'm beginning to understand the concept of linked lists and structs but am a little unclear on the syntax. From what I gather so far,
struct node
{
int data; //data of variable
struct *next; //points to the next node in the structure
}
I'm unsure on how to write the syntax for the list.
Ultimately I want to create a 5 by 5 board for a wordsearch and have the list go through each element to check if the user inputs matched in of the possible words and then output a response; then update the table for the next round. (Basically create a very very simple word search). Thanks!
2 AnswersProgramming & Design7 years agoWhen using Linked Lists in C Programming?
So I'm beginning to understand the concept of nodes and linked lists but am confused about something. Say I have a table 3 by 3 and I want to go through each cell to check what the value is. From what I understand, to create a node, i would have to do the following:
struct NODE{
int data; //stores data of node
NODE* next; //points to the next node
}
I'm not sure if that's completely right. My question is, do I need a seperate node for each element (so would that be 9 different nodes?). If so, I can't imagine I would have to write the above 9 times over so how would i create this linked list (in simple terms). Thanks!
3 AnswersProgramming & Design7 years agobinary trees in C programming?
I'm new to binary trees and am confused with how to do the following. If you could offer some advice or guidance (in the simplest way to understand), that would be appreciated greatly.
I have the following
20(10(5(*)(*))(17(*)(*)))(30(21(*)(*))(*))
I want to write a program that reads in a binary tree in the above form and displays it in a 'friendlier' style
20----30
| |
10-17 21
|
5
The binary tree is read in using argv[1](this is the string itself, NOT a filename), for example :
% treevis "20(*)(*)"
Could you explain what I have to do for this and how I should go about doing it. My knowledge of trees is very raw at the moment so any advice would be great. Thanks!
2 AnswersProgramming & Design7 years agoDifference between Linked Lists and Queus in C?
What is the difference and when should they be used?
1 AnswerProgramming & Design7 years agoWhat is recursion in C used for?
From what I understand, It's a functions within itself. However I don't understand what the purpose of this is and why it's used. Could you explain why and when this should be used (other than to find out the factorial of a number)? What are the different things I need to know about recursion? Thanks!
2 AnswersProgramming & Design7 years agoQuestion about Pointers in C programming?
So I'm trying to understand pointers and so far I've gotten that a pointer is a variables that points to the memory address if another variable. That memery address is the pointers variables' value. Is that correct? So:
int a;
int *ptr;
ptr = &a;
Though I don't understand a few things. Firstly what is point in having a NULL pointer is it isn't going to point to anything? Secondly what are pointers generally used for when writing code? I've only been writing simple code so far. Could you further explain why and how pointers may be used (preferably in the simplest way to understand). Thanks!
2 AnswersProgramming & Design7 years agoLinked lists and 2D arrays in C?
If I had board with 5 rows and 5 columns, how would I use linked lists to check each row for it's current value. I want to create a board which looks like
. . O . .
. . O . .
OOOOO
. . O . .
. . O . .
The dots represent empty cells and the O's represent little checkers pieces. The pieces can only move if the jump a neighboring piece and land on an empty cell (as in checkers). I know how to print a board but I need to write something that would show all the possible moves the board would make. I don't want any user input (other than maybe start game) . Any hints on how I would do this?
ps: Only one piece can move per round and obviously the table will change with every round, until there are no/or one peg left.
1 AnswerProgramming & Design7 years ago3 basic questions about C programming?
I had a few basic questions about C.
1)Why and when is 'int main(int argc, char *argv[])' instead of 'int main'
2)When creating a function, when should you use 'void 'function name'' and what is the different between using int ''function name'? Basically I don't know why some functions are void and some are int
3)Could you explain 'return' to me. I've seen you can have return 0 or return 1 or return void, etc. What is the difference
3 AnswersProgramming & Design7 years agoHow to you create and print a table in C?
I've been learning C and one of the things that I have struggled with is creating and printing tables/grids/boards. I have an idea that it will require 2d arrays, linked lists and a few functions but am unsure as to what the simplest way to do this is. From what I think I understand so far:
//your basic libraries
#include <stdio.h>
#include <stdlib.h>
#define ROWS 10
#define COLS 10
void board();
void printboard();
//
int main(int argc, char *argv[])
{
return
}
//
void board()
{
int board[ROWS][COLS]
int i, j;
for(i = 0; i < ROWS; i++)
for(j = 0; j < COLS; j++)
}
//unsure how to do this
void printboard()
{
}
That's what I understand so far. Could you correct my mistakes and explain how to do this as simply as possible. Much appreciated!
Programming & Design7 years agoHow would a design a 4 bit ALU?
That commutes the following functions
AB (bit by bit AND)
A+B (bit by bit OR)
A XOR B " "
Sum of A and B
It's the last problem I'm stuck on. I have a vague idea on what to for the ALU but am struggling on the functions.
1 AnswerProgramming & Design7 years agoState diagram for Vending machine?
I have had a go but need to be sure. The question says that it can only accept 10p, 20p, 50p (one coin at a time). If the user exceeds the cost of the soda (which is 50p), the money will be returned. Any hints on what I should do?
1 AnswerProgramming & Design7 years agoDesigning a 4-bit register?
What steps do I need to take to complete the following question
Design a 4-bit register with 3 control inputs s2,s1 and s0, data inputs I3,I2,I1, and I0, and 4 data outputs Q3, Q2, Q1, and Q0. When s2s1s0=000 the register maintains it value. When (a) s2s1s0=001 the value is unchanged (b) s2s1s0=010, the register shifts left by one bit (c) s2s1s0=011 the register shifts the value to right,(d) s2s1s0=100 it loads the right rotate shift of the value stored in the register an if s2s1s0=101 it loads the left rotate shift of the contents of the register. Finally if s2s1s0=110 it loads the complement of the value stored in the register.
I haven't done this before. Would I need to start off with a truth table? What else would I need to do. If you could go into as much detail as possible, that would be great. Cheers!
1 AnswerProgramming & Design7 years agoCalculating gate delay for 4-bit multiplier?
The multiplied has 16 2 input AND gates (with a gate delay of 1ns),
4 half adders (with a gate delay of 2ns)
and 8 full adders (with a gate delay of 3ns)
to answer this question, would I simple just had all the delays together (so that would be 16 + 8 + 24 = 48ns). The question asks to estimate the total gate delay of the multiplication
4 AnswersProgramming & Design7 years agoCalculating gate delay for 4-bit multiplier?
The multiplied has 16 2 input AND gates (with a gate delay of 1ns),
4 half adders (with a gate delay of 2ns)
and 8 full adders (with a gate delay of 3ns)
to answer this question, would I simple just had all the delays together (so that would be 16 + 8 + 24 = 48ns). The question asks to estimate the total gate delay of the multiplication
1 AnswerEngineering7 years agoHow to write the following code in c?
My current topic is with lists and recursions which I don't quite understand. The assignment has to to with the pattern locks that you get on most mobile phones where you can unlock a phone by moving up, down, left, right, etc (but not diagonally). The things that I have to do are the following.
1)write a code for a pattern lock (doesn't have to be complicated. Simple is good)
2)find out how many possible combinations the user can have (assuming that the possible numbers are 1-9)
3)how many different are available on a 3 by 3 grid if the user starts on the top left corner, the bottom left, middle left
Any advice on this would be immensely appreciated.
1 AnswerProgramming & Design7 years agoHow to talk to a cute girl who catches the same train?
There's this girl who I think is really pretty and catches the same train as me. Upon arriving at the station, I would wait walk about 50 yards and wait at the far end. Today she when she came, she did the same thing and stood about a meter away from me. After getting on the train, I sat across her about 1 yard away but we both had out headphones in. I caught her looking at me a few times, so she might be interested.
How exactly should I break the ice with her? It's not like at a bar where it's much easier to talk to someone. This is a public train station/train. I know that after the ice is broken, I will have no problem at all but what could I say to this 'stranger' that wouldn't freak her out or startle her? She did seem quite shy.
4 AnswersSingles & Dating8 years agoAny recent teen slasher films?
Feel like watching a teen slasher horrer movie. Can you name some that have been released in the last few years please?
1 AnswerMovies8 years agoWhat to do a PHD study in?
Received undergrad in Advanced Maths, MA in Computer Science. What would be a good next step as I'm convinced I want to further study. What possible courses should I look at and why would that be a good step. Thanks!
1 AnswerHigher Education (University +)8 years ago