Yahoo Answers is shutting down on May 4th, 2021 (Eastern Time) and beginning April 20th, 2021 (Eastern Time) the Yahoo Answers website will be in read-only mode. There will be no changes to other Yahoo properties or services, or your Yahoo account. You can find more information about the Yahoo Answers shutdown and how to download your data on this help page.
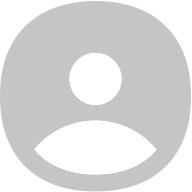
bigboss
python programming help?
i need to implement a way in python to tunnelling IP over DNS,
how do i do that?
where should i start?
what should i look at?
please help me i really dont know where to start
3 AnswersProgramming & Design8 years agotunnelling IP over DNS discuss?
i have a exam in computer networks and I know this question is coming up, help please, i dont really know how to start
1 AnswerComputer Networking8 years agowhat can i do diffrently to slim down my python code?
!/usr/bin/python
import sys
import os
#the edge object holds information about an edge, that is, a vector joining two nodes on the network
class Edge(object):
def __init__(self, fromSource, toSink, capacity):
self.source = fromSource
self.sink = toSink
self.capacity = capacity
def __repr__(self):
return self.fromSource.name.strip() + " - " + self.toSink.name.strip()
class FlowNetwork(object):
def __init__(self):
self.adj = {}
self.flow = {}
# add a vertes to the known list of verteces
def add_vertex(self, vertex):
self.adj[vertex] = []
def get_edges(self, toSink):
return self.adj[toSink]
# add information about an edge to the known network topography
def add_edge(self, fromSource, toSink, capacity=0):
if fromSource == toSink:
raise ValueError("fromSource == toSink")
edge = Edge(fromSource,toSink,capacity)
redge = Edge(toSink,fromSource,0)
edge.redge = redge
redge.redge = edge
self.adj[fromSource].append(edge)
self.adj[toSink].append(redge)
self.flow[edge] = 0
self.flow[redge] = 0
# seraches for a path fromsource to sink
def find_path(self, source, sink, path):
if source == sink:
return path
for edge in self.get_edges(source):
residual = edge.capacity - self.flow[edge]
if residual > 0 and not (edge,residual) in path:
result = self.find_path( edge.sink, sink, path + [(edge,residual)] )
if result != None:
return result
# given a source and a sink within the knwon network topograhpy,
# uses the ford fulkerson algorithim to find the maximum flw between source and sink
def max_flow(self, source, sink):
print("finding path from %s to %s" % (source, sink))
path = self.find_path(source, sink, [])
while path != None:
print(path)
flow = min(res for edge,res in path)
for edge,res in path:
self.flow[edge] += flow
self.flow[edge.redge] -= flow
path = self.find_path(source, sink, [])
return sum(self.flow[edge] for edge in self.get_edges(source))
1 AnswerProgramming & Design8 years agowhat can i do diffrently to slim down my python code?
class Edge(object):
def __init__(self, u, v, w):
self.source = u
self.sink = v
self.capacity = w
def __repr__(self):
return "%s->%s:%s" % (self.source, self.sink, self.capacity)
class FlowNetwork(object):
def __init__(self):
self.adj = {}
self.flow = {}
def add_vertex(self, vertex):
self.adj[vertex] = []
def get_edges(self, v):
return self.adj[v]
def add_edge(self, u, v, w=0):
if u == v:
raise ValueError("u == v")
edge = Edge(u,v,w)
redge = Edge(v,u,0)
edge.redge = redge
redge.redge = edge
self.adj[u].append(edge)
self.adj[v].append(redge)
self.flow[edge] = 0
self.flow[redge] = 0
def find_path(self, source, sink, path):
if source == sink:
return path
for edge in self.get_edges(source):
residual = edge.capacity - self.flow[edge]
if residual > 0 and not (edge,residual) in path:
result = self.find_path( edge.sink, sink, path + [(edge,residual)] )
if result != None:
return result
def max_flow(self, source, sink):
path = self.find_path(source, sink, [])
while path != None:
flow = min(res for edge,res in path)
for edge,res in path:
self.flow[edge] += flow
self.flow[edge.redge] -= flow
path = self.find_path(source, sink, [])
return sum(self.flow[edge] for edge in self.get_edges(source))
1 AnswerProgramming & Design8 years agowhat is automated penetration test?
what is the difference between automated penetration test and manual if that's the word to use it?
what are some tools to perform automated and some to perform manual, or are the same tools but set differently
5 AnswersSecurity8 years agopython programming help, im trying to save the scan into a file which allows the user to choose the file name?
hi this is my code i want to save the file after the scan is finish into a txt file, can anyone help please
#!/usr/bin/python
#Color For Terminal
r = '\033[31m' #red
b = '\033[34m' #blue
g = '\033[32m' #green
print g + "hello there"
print ""
print b+ "##################################################"
print "# quicksnap - A Customized Nmap Automatic Scanner#"
print "##################################################"
print ""
print r+ "Scanning Options"
print "[1] Intense Scan"
print "[2] Intense Scan + UDP"
print "[3] Intense Scan - all TCP ports"
print "[4] Intense Scan w/out ping"
print "[5] Ping Scan"
print "[6] Quickie Scan"
print "[7] Quick Traceroute"
print "[8] Normal Scan"
print "[9] Send Bad Checksums"
print "[10] Generate Randon Mac Adress Spoofing for Evasion"
print "[11] Fragment Packets"
print "[12] Check for Possible Vulnerabilities"
option = raw_input("Choose your Scanning Option:")
print g+ ""
if option == '1':
ip = raw_input("Input IP Address / Hostname:")
os.system("nmap -T4 -A -v "+ip)
elif option == '2':
ip = raw_input("Input IP Address / Hostname:")
os.system("nmap -sS -sU -T4 -A -v "+ip)
elif option == '3':
ip = raw_input("Input IP Address / Hostname:")
os.system("nmap -p 1-65535 -T4 -A -v "+ip)
elif option == '4':
ip = raw_input("Input IP Address / Hostname:")
os.system("nmap -T4 -A -v -Pn "+ip)
elif option == '5':
ip = raw_input("Input IP Address / Hostname:")
os.system("nmap -sn "+ip)
elif option == '6':
ip = raw_input("Input IP Address / Hostname:")
os.system("nmap -T4 -F "+ip)
elif option == '7':
ip = raw_input("Input IP Address / Hostname:")
os.system("nmap -sn --traceroute "+ip)
elif option == '8':
ip = raw_input("Input IP Address / Hostname:")
elif option == '9':
ip = raw_input("Input IP Address / Hostname:")
os.system("nmap --badsum "+ip)
elif option == '10':
ip = raw_input("Input IP Address / Hostname:")
os.system("nmap -sT -Pn --spoofmac 0 "+ip)
elif option == '11':
ip = raw_input("Input IP Address / Hostname:")
os.system("nmap -f "+ip)
elif option == '12':
ip = raw_input("Input IP Address / Hostname:")
os.system("nmap --script vuln "+ip)
else:
print b+ "Your Choice is Invalid"
print "Goodbye"
name_of_file = raw_input("write file name: ")
completeName = name_of_file + ".txt"
3 AnswersProgramming & Design8 years agopython code to find the max flow of ford-fulkerson algorithim?
can anyone help me on how to find the max flow of a network using the ford-fulkerson algorithim, i need to do that in python and im not very good in programing, all my attempts goes wrong
i check the code on wikipedia but still no joy
help anyone please
2 AnswersProgramming & Design8 years agopython help please understanding the code?
!/usr/bin/env python
from socket import *
if __name__ == '__main__':
target = raw_input('Enter host to scan: ')
targetIP = gethostbyname(target)
print 'Starting scan on host ', targetIP
#scan reserved ports
for i in range(20, 1025):
s = socket(AF_INET, SOCK_STREAM)
result = s.connect_ex((targetIP, i))
if(result == 0) :
print 'Port %d: OPEN' % (i,)
s.close()
i just took this code from the internet to scan for open ports, i put into python idle the code run fine but i dont understand the code, i can understand the output is pretty clear but i cant understand the code
this is the output:
Enter host to scan: localhost
Starting scan on host 127.0.0.1
Port 135: OPEN
Port 445: OPEN
can anyone explain this code for me please
how could i put coments to understand this code better?
thanks
2 AnswersProgramming & Design8 years agopython line of code to store the output as an xml file?
!/usr/bin/env python
from socket import *
if __name__ == '__main__':
target = raw_input('Enter host to scan: ')
targetIP = gethostbyname(target)
print 'Starting scan on host ', targetIP
#scan reserved ports
for i in range(20, 1025):
s = socket(AF_INET, SOCK_STREAM)
result = s.connect_ex((targetIP, i))
if(result == 0) :
print 'Port %d: OPEN' % (i,)
s.close()
this is my code, how can i tell python to writte this document to a file as an .xml file on a folder on my desktop
1 AnswerProgramming & Design8 years agopython script to scan for sql servers?
how can i scan for sql servers with python
2 AnswersProgramming & Design8 years agopython script to scan the local host?
simple script to scan my local host with python
127.0.0.1
2 AnswersProgramming & Design8 years agoin computer network what is fingerprinting?
2 AnswersComputer Networking8 years agowhat is cybersquatting?
how to improve doman name protection in U.K regarding cybersquatting
3 AnswersWords & Wordplay8 years agohow to improve domain name protection in the UK?
in the context of cyber squatting
can anyone suggest some quality source where i could check that as well
thanks
1 AnswerOther - Education8 years agohow does court action work regarding statutory law?
how case laws deal with issues relating to domain names, and what are the advantages of taking such action
3 AnswersLaw & Ethics8 years agohow to import xml documentos using pyhton to read the data from it?
i have a xml document that is an output of a nmap scan, now I'm trying to import into python to be able to read the file by different elements for example:
<?xml version="1.0"?>
<data>
<country name="Liechtenstein">
<rank>1</rank>
<year>2008</year>
<gdppc>141100</gdppc>
<neighbor name="Austria" direction="E"/>
<neighbor name="Switzerland" direction="W"/>
</country>
<country name="Singapore">
<rank>4</rank>
<year>2011</year>
<gdppc>59900</gdppc>
<neighbor name="Malaysia" direction="N"/>
</country>
<country name="Panama">
<rank>68</rank>
<year>2011</year>
<gdppc>13600</gdppc>
<neighbor name="Costa Rica" direction="W"/>
<neighbor name="Colombia" direction="E"/>
</country>
</data>
if im using this file and i just want to print the countries i can do something like:
>>> for country in countr:
... print country.get('name')
...
Liechtenstein
Singapore
Panama
____________________________________________
I'm able to do most of the thing with the element tree, like find, finfall, etc, my main problem is how to import my file into python to be able to read the data
the file is in: C:/Users/Heleno/Desktop/results/primeiro.xml
im trying to do the tutorial:
http://docs.python.org/2/library/xml.etree.element...
but still dont understand how they manage to import the file.
the bit of code i have:
import xml.etree.ElementTree as ET
tree = ET.parse('primeiro.xml')
root = tree.getroot()
i put this into python idle but i think i need to be more specific with the root where the file is
1 AnswerProgramming & Design8 years agohow to create a project plan?
I did my proposal for my project in uni but now my project supervisor say that I need a project plan with milestone of the project, how do I do that what should I include in a project plan
1 AnswerProgramming & Design9 years agowhy LAN health check software use xml language?
whats the end of using the xml
is it to allow the configuration of the files?
2 AnswersProgramming & Design9 years agohow to develop a tool to manage social netwoking sites?
how to develop a tool to manage social netwoking sites, are there any tools/software available
1 AnswerGoogle9 years ago