Yahoo Answers is shutting down on May 4th, 2021 (Eastern Time) and beginning April 20th, 2021 (Eastern Time) the Yahoo Answers website will be in read-only mode. There will be no changes to other Yahoo properties or services, or your Yahoo account. You can find more information about the Yahoo Answers shutdown and how to download your data on this help page.
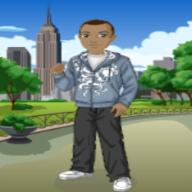
gamer3425
Java Assignment Help?
The prompt is as follows:
Write a program that prompts the user to enter the number of students in a class, the student names and their scores on a test. The program should print the student names (along with their scores) in decreasing order of their scores (use the selection sort as covered in class). Your sort should be implemented as a method (please see the following method header):
Here is my code:
public class Assignment5 {
/**
* @param args
*/
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.println("Enter the size of the class: ");
int size = input.nextInt();
String[] names = new String[size];
double[] scores = new double[size];
for (int i = 0; i < size; i++) {
System.out.print("Please enter a student name: ");
input.useDelimiter(System.getProperty("line.separator"));
names[i] = input.next();
System.out.print("Please enter " + names[i] + "'s score: ");
scores[i] = input.nextDouble();
}
System.out.println("The class size is " + size);
SelectionSort(scores, names);
}
public static void SelectionSort (double[] score, String[] name) {
Scanner myInput = new Scanner(System.in);
for (int i = 0; i < score.length; i++) {
// This finds the minimum in the list.
double currentMin = score[i]; // Minimum = position in the list.
int currentMinIndex = i; // Index of the current minimum.
for (int j = i + 1; j < score.length; j++) {
if (currentMin < score[j]) {
currentMin = score[j];
currentMinIndex = j;
}
}
if (currentMinIndex != i) {
score[currentMinIndex] = score [i];
score[i] = currentMin;
}
}
System.out.println("Name Score");
for (int i = 0; i < score.length; i++) {
System.out.print(name[i] + score[i]);
System.out.println();
}
}
}
Now the problem is that when it does the sort, it doesn't put the grade with its associated score. What do I do to correct this problem?
3 AnswersProgramming & Design8 years agoJava Programming Homework Help?
The assignment is to create a class called MyInteger that contains the following:
• An int data field named value that stores the int value of an integer.
• A constructor that creates a MyInteger object for the specified int value.
• A get method that returns the int value.
• A method, isPrime() that returns true if the value is a prime number. See section 4.10 of the text for Java code that detects prime numbers (this may vary depending on the edition you have).
• A static, isPrime(MyInteger) that returns true if the value is a prime number. Note that this method takes an object reference variable (rather than the value) as a parameter.
The output should look like this:
Testing Instance method, is Prime
isPrime: 997 is prime
-----------------------------------
Testing Class method (that takes a reference variable)is Prime
isPrime: 997 is prime
Testing Instance method, is Prime
isPrime: 998 is not prime
-----------------------------------
Testing Class method (that takes a reference variable)is Prime
isPrime: 998 is not prime
My code is as follows:
public class Assignment4 {
/**
* @param args
*/
static boolean isPrime(int value) {
boolean prime = true;
//check to see if the number is prime
for(int j=2; j <= value / 2; j++)
if (value % j == 0) {
prime = false;
}
return prime;
}
public static void main(String[] args) {
MyInteger number = new MyInteger(997);
if (isPrime(997)) {
System.out.println(number + " is prime.");
}
}
class MyInteger {
int value = 1;
MyInteger() {
this(1);
}
MyInteger(int number) {
value = number;
}
public boolean isPrime() {
boolean prime = true;
//check to see if the number is prime
for(int j=2; j <= value / 2; j++)
if (value % j == 0) {
prime = false;
}
return prime;
}
// Accessor that returns value.
public int getValue() {
return value;
}
}
}
What am I doing wrong?
1 AnswerProgramming & Design8 years agoHELLLLLLLLLLLLLLLLLLLLP?
Is there a way to install a program like Zune in the administrator account without it being installed to the other standard accounts?
2 AnswersSoftware1 decade agoHEEEEEEEEEEEEEEEEEELP?
A long time ago, there was a video game created with a young boy and his girlfriend Tabby. I can't remember the name of this game for the life of me. It was on playstation and I played it on a jampack disc. WHAT WAS IT CALLED
1 AnswerVideo & Online Games1 decade agoQUESTION ABOUT NFL!!!?
There used to be someone on the starting lineups. When he said his name, he made it seem like his name was really long. Who was it?
5 AnswersFootball (American)1 decade agoMP3 PLAYER ISSUES!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!?
I have a philips gogear mp3 player. The model is SA3285/37. When I turn it on, the play button won't work. When I try to choose a song, it shuts itself off. It doesn't even shut off all the way! The only was I can shut it off is by resetting it and plugging it in to charge. What should I do?
2 AnswersMusic & Music Players1 decade agoMy document and windows media player closes by themselves. I tried to do system restore, but the problem comes?
My documents and windows media player closes by themselves. I tried to do system restore, but it would fix the problem for about thre days then the problem comes back. WHAT DO I DO?
2 AnswersOther - Computers1 decade agoComputer help??????????????????????????????
My Documents recognizes mp3 files, but I can't input info such as the artists name or cd title. What do I do?
2 AnswersSecurity1 decade agoMovies with the literary technique aside?
Any movies with aside or soliloquy?
2 AnswersWords & Wordplay1 decade agoEVERYBODY SING!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!?
Those of you who are mad at me, the song is called annoying song.
http://www.youtube.com/watch?v=gMTeytJeSp8
Now that you know, SING!!!
Oh my word this tune is annoying yes i know its really annoying but i can't get this song out of my he-ead.
2 AnswersSinging1 decade agoLG 300 PHONE QUESTION ?
Anyone know EXACTLY how to get ringtones for an LG 300 NET10 phone. IF YOU HAVE THE PHONE, PLEASE TELL ME.
1 AnswerCell Phones & Plans1 decade agoWhich Nintendo Wii games will help me lose the most weight?
A list would be very helpful. Any and all answers will be accepted.
4 AnswersVideo & Online Games1 decade agoHELP ME!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!?
I'm around 5'6, and weigh 216. am I overweight? If so, what should I do to lose weight. I tried working out and am getting no results. I've been working out for a few weeks now. What do I do?
4 AnswersDiet & Fitness1 decade agoDATING ADVICE FOR FRESHMAN!?
I know it seems kind of early, but I'm a freshman in high school and I really want a girlfriend. I am thinking of playing football just to be noticed by girls. I'm not the most handsome guy, but I'm faithful. How do I get girls? I weigh 210 pounds and I am kind of athletic. Should I lose weight?
4 AnswersSingles & Dating1 decade agoDating advice for freshmen?
It's me again.. Just so you'll know, I only weigh 210. I'm not like 350 pounds or anything like that. But in all girls' eyes, I'm fat.
1 AnswerSingles & Dating1 decade agoDating advice for freshman?
Does anyone know how a fat, ugly freshman can get a girlfriend? all of the girls at my school only date skinny guys and it hurts a lot......Help me please....I'm desperate for answers.......
8 AnswersSingles & Dating1 decade ago