Yahoo Answers is shutting down on May 4th, 2021 (Eastern Time) and beginning April 20th, 2021 (Eastern Time) the Yahoo Answers website will be in read-only mode. There will be no changes to other Yahoo properties or services, or your Yahoo account. You can find more information about the Yahoo Answers shutdown and how to download your data on this help page.
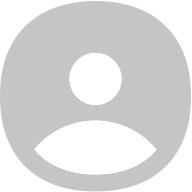
sesh48
I am in desperate need of help with this C code and why it fails to work..?
I am trying to get the C program to work. Whole point of the program is that its supposed to check the strings and reorder them in alphabetical order while ignoring any non alphabetic characters within the strings. My program seems to not do it totally right. Someone help out and it'd be really nice of you.
#include <stdio.h>
#include <stdlib.h>
#include <errno.h>
#include <math.h>
#include <string.h>
void vowel (const char * string);
void reorder (int n, char *x[]);
int main () /* Main Function */
{
const char * random = "\nThis is a test run."; /* Const Char string passed as variable random */
printf(random, "%s"); /* Output Statement of 'random' */
vowel(random); /* Calling function 'vowel' */
const char * myname = "\nMy name is Shiva Devarinti."; /* Const Char string passed as variable myname */
printf(myname, "%s"); /* Output Statement of 'myname' */
vowel(myname); /* Calling function 'vowel' */
const char * university = "\nI go to Binghamton University."; /* Const Char string passed as variable university */
printf(university, "%s"); /* Output Statement of 'university' */
vowel(university); /* Calling function 'vowel' */
static char *x[2] = { "Tr2o3phy","Sho2e"}; /* Declaration of array of two pointers */
static char *y[2] = { "Artificial","Art"};
static char *z[2] = { "zoom","Shoe"};
int i;
printf ("\nThe entered strings are:\n"); /* Outputs the entered strings */
for (i = 0; i < 2; ++i)
{
printf ("%s\n", x[i]);
}
printf ("\nThe entered strings are:\n");
for (i = 0; i < 2; ++i)
{
printf ("%s\n", y[i]);
}
printf ("\nThe entered strings are:\n");
for (i = 0; i < 2; ++i)
{
printf ("%s\n", z[i]);
}
int n = 2;
reorder (n, x);
reorder (n, y);
reorder (n, z); /* Sort and reorder the list of strings */
printf("\nThe reordered string is:\n");
for (i = 0; i < n; ++i)
{
printf("\n %d: %s\n", i + 1, x[i]); /* Display the reordered list of strings */
}
printf("\nThe reordered string is:\n");
for (i = 0; i < n; ++i)
{
printf("\n %d: %s\n", i + 1, y[i]);
}
printf("\nThe reordered string is:\n");
for (i = 0; i < n; ++i)
{
printf("\n %d: %s\n", i + 1, z[i]);
}
return 0;
}
void vowel (const char * string) /* Function declaration */
{
int count = 0; /* Variable 'count' Declaration and Initialization */
int store = 0; /* Variable 'store' Declaration and Initialization */
while ( string[count] !='\0') /* Beginning of while loop */
{
/* If an upper case or lower case vowel is encountered add to the variable 'store' and increment it */
if (string[count] == 'A')
++store;
if (string[count] == 'a')
++store;
if (string[count] == 'E')
++store;
if (string[count] == 'e')
++store;
if (string[count] == 'I')
++store;
if (string[count] == 'i')
++store;
if (string[count] == 'O')
++store;
if (string[count] == 'o')
++store;
if (string[count] == 'U')
++store;
if (string[count] == 'u')
++store;
++count;
}
printf ("\nThe Number of vowels in the above sentence are %i.\n", store); /* Output Statement */
}
void reorder (int n, char *x[])
/* Function Declaration */
{
char *temp; /* Variable Declaration */
int i, item;
for (item = 0; item < n - 1; ++item) {
for (i = item + 1; i < n; ++i) {
if (strcmp (x[item], x[i]) > 0) { /* Swapping the strings */
temp = x[item];
x[item] = x[i];
x[i] = temp;
}
}
}
return;
}
2 AnswersProgramming & Design1 decade agoI need some help with the following C code.?
I am trying to do a string compare and swap the strings in order to print them in alphabetical order.
Here is my code:
#include <stdio.h>
#include <stdlib.h>
#include <errno.h>
#include <math.h>
#include <string.h>
int main (void)
{
static char *x[2] = {"Hello",
"Bye" };
int i;
for (i = 0; i < 2; ++i)
printf ("%s\n", x[i]);
printf ("\n");
int n = 2;
reorder (n , x);
return 0;
}
void reorder(int n, char *x[])
{
char *temp;
int i, item;
for (item = 0; item < n - 1; ++item)
for (i = item + 1; i < n; ++i)
if (strcmp(x[item], x[i]) > 0) {
temp = x[item];
x[item] = x[i];
x[i] = temp;
printf(temp);
}
return;
}
4 AnswersProgramming & Design1 decade agoI'm having some trouble with my C code and I keep having the same error repeatedly.. Any help would be great..?
I keep having the same repeated error of "Segmentation Fault" at the end of running the program.
My code is as follows:
#include <stdio.h>
#include <stdlib.h>
#include <errno.h>
#include <math.h>
#include <string.h>
main ()
{
int i, n = 0;
char *x[10];
printf("Enter each string on a separate line below\n\n");
printf("Type \'END\' when finished\n\n");
do {
x[n] = (char *) malloc(12 * sizeof(char));
printf("string %d: ", n + 1);
scanf("%s", x[n]);
}
while (strcmp(x[n++], "END"));
/* reorder the list of strings */
reorder(--n, x);
/* display the reordered list of strings */
printf("\n\nReordered List of STrings:\n");
for (i = 0; i < n; ++i)
printf("\nstring %d: %s", i + 1,x[i]);
}
void reorder(int n, char *x[])
{
char *temp;
int i, item;
for (item = 0; item < n - 1; ++item)
for (i = item + 1; i < n; ++i)
if (strcmp(x[item], x[i]) > 0) {
temp = x[item];
x[item] = x[i];
x[i] = temp;
}
return;
}
3 AnswersProgramming & Design1 decade agoI've a question about Programming in the language C..?
I am trying to write a basic program in C and as I am a beginner I could use some help. I need to learn this material fast and I'm having a lot of trouble. If someone could help me out with the following that'd be great.
Everything below is supposed to be done with basic C commands only which is restricted to strings, arrays, loops such as for and if loops, and pointers and basic commands like that. I am really lost and would appreciate some code help.
1.
Create a new C function which will count the number of vowels (A, E, I, O or U, capital or lower case) in a string passed to it, and return that number (as an "int"). Note that the type of the string passed into this function should be "const char *" - as strings are really arrays of "char"s - which, in turn, are really constant pointers to "char"s).
Add to your main program several test calls to this function, and print out the strings passed in and the responses to the screen. Be sure to check any corner cases you can think of.
2.
Add another C function to your program which returns nothing (void), but takes two writable strings and compares them. If the first is alphabetically (see here) after the second, then your function should completely swap the contents of the two strings before returning. It is the caller's responsibility to be sure the memory the strings reside in is writable and that there is enough space in the array holding each to hold the other should they need to be swapped (and you should note these limitations in the comments for this function).
Note that this sort should NOT be case-sensitive (so neither "Ape" nor "ape" is alphabetically after the other). Non-letters should be entirely ignored and skipped (so neither "ape" or "432a--P. E++" is alphabetically after the other). Also, shorter words should be sorted before longer words which contain that entire shorter word at their beginning (so "fish" comes before "Fisher").
Add to your main program several tests of this, outputting the results of each.
3 AnswersProgramming & Design1 decade agoapartment lease question.. Forgot to mention details in previous one.?
I am currently living in an apartment complex and I just moved in a few days ago. I am totally not getting along with my apartment mate and even if I lose my deposit I want to move out. Problem is when I asked the superintendent what the terms of the lease were she said there was no way of breaking it and that even if I leave I would have to pay each month's rent for the rest of the way. Is that actually true or she just bluffing me and trying to make me stay because I am a college student?
5 AnswersRenting & Real Estate1 decade agoDo dinosaurs laugh?
curious as to laughter amongst dinosaurs. does it actually exist or do they just roar?
6 AnswersZoology1 decade agoI've a physics question posted below if someone could help me with it or answer it for me that'd be great..
As you look out of your dorm window, a flower pot suddenly falls past. The pot is visible for a time t , and the vertical length of your window is Lw. Take down to be the positive direction, so that downward velocities are positive and the acceleration due to gravity is the positive quantity g.
Assume that the flower pot was dropped by someone on the floor above you (rather than thrown downward).
From what height above the bottom of your window was the flower pot dropped?
Express your answer in terms of Lw,t , and g .
thanx for any help
2 AnswersPhysics1 decade agohow do i downlaod and install an antivirus file and key from a torrent??
the antivirus plus key is a .rar file and i'm trying to download it so i'm wondering how i download and install it right.. thanx for ur answers..
2 AnswersOther - Computers1 decade agoI've a few quick chem questions if someone could help me out with them that'd be great..?
Balance the following equation for a reaction in acidic solution. Only H+ or H2O may be added. Enter your answer as the sum of the coefficients.
HgS(s) + Cl-(aq) + NO3- --> HgCl42-(aq) + NO2(g) + S(s)
The following reaction occurs in basic solution. Balance it by adding only OH- or H2O. Enter your answer as the sum of the coefficients.
Mn2+(aq) + MnO4-(aq) --> MnO2(s)
Oxygen gas can be generated by heating KClO3 in the presence of some MnO2.
2KClO3 --> 2KCl + 3O2
If all the KClO3 in a mixture containing 2.31 g KClO3 and 0.35 g MnO2 is decomposed, how many liters of O2 gas will be generated at T = 21.0oC and P = 758.8 torr?
1 AnswerChemistry1 decade agook I've a quick question for all you geometry people out there.. question below:?
5. [1pt]
Which of the following shapes have 5-fold rotational geometry? (Give ALL correct answers: B, AC, BCD..)
A) a stop-sign (ignore writing)
B) an equilateral triangle
C) the letter X
D) a cube
E) a soccer ball
F) a water molecule (hint: H-O-H is a bent molecule)
there can be any number of answer choices and it'd be great if someone could explain what a five fold rotational geometry actually is..
1 AnswerMathematics1 decade agoI've a quick chem question if someone could help me out that'd be great.. question below:?
The second-order Bragg reflection of x-radiation with l=1.660Å from a set of parallel planes in copper occurs at angle 2q=54.90o. Calculate the distance between scattering planes of the crystal in Å.
1 AnswerChemistry1 decade agoHow do I convert a movie from avi to whatever format I need to watch it on my TV with a dvd player??
Two questions in this: what format works with a regular DVD player like on a tv?? I kno avi is just for computers so what do I convert it to?? Second off whats the best software to convert it??
6 AnswersSoftware1 decade agoI've a quick chem question if someone can help me understand this it'd be great.. thanx..?
The second-order Bragg reflection of x-radiation with l=1.660Å from a set of parallel planes in copper occurs at angle 2q=54.90o. Calculate the distance between scattering planes of the crystal in Å.
1 AnswerChemistry1 decade agoanother chem question any explanations and answers would be great.. question below:?
Manometers can use liquids other than mercury to measure small pressure differences. On a day when the barometric pressure is 763.0 torr, a water manometer is connected between the atmosphere and a glass bulb containing a sample of helium gas has a height difference of 30.2 cm, The water level is higher in the arm connected to the bulb. Calculate the pressure in the bulb (in torr).
thanx for any responses guys..
1 AnswerChemistry1 decade agohey everyone I've a quick chem question its part of my hw and i'm struggling with it.. question below..
if anyone can explain it and answer it for me.. even if its just an explanation as to how to do it that'd be great guys thanx..
here's the question:
Liquid helium at 4.2 K has a density of 0.147 g/mL. Suppose that a 2.50-L metal bottle at 90K contains air at 3.0 atm pressure. If we introduce 100.0 mL of liquid helium, seal the bottle, and allow the entire system to warm to room temperature (25oC), what is the pressure inside the bottle (in atmospheres)?
1 AnswerChemistry1 decade agoI need some help with overclocking a pc if you guys could help me that'd be great.. details below:?
I want to overclock my pc which is a custom built pc.. i built it myself a summer ago but now it doesn't feel so fast anymore so i wanna overclock it.. I've a AMD athlon 64 3700 processor and the standard heatsink that comes with it. I've 512 ram on it and windows XP.. the AMD works wonders on games and game graphics but if it worked a lil faster would be great.. right now it runs at bout 2.3 ghz.. i was thinkin if i could push like 2.6 or so on it.. any suggestions would be great.. also how do u overclock ram?? again any suggestions r appreciated.. thanx guys..
2 AnswersDesktops1 decade agohow do i overclock the processor on my compaq presario F572US laptop??
So i got a laptop for college and its a really cheap 450 dollar compaq presarion laptop.. i've a dual core AMD athlon 64 x2 on it and it runs like 1.73ghz i think.. i wanna overclock it so it runs faster.. i've windows vista and a gig or ram on it although i dunno if either of them have anything to do with overclocking but just mentioning them.. btw i don't wanna kill my laptop so i don't wanna open it up.. if there's like any easy way like messin with the bios r somethin that'd work.. well thanx for any answers guys..
5 AnswersLaptops & Notebooks1 decade agoif any of u guys could help me with some of my chem lab questions that'd be great.. look below:?
i'm really struggling with chem and if anyone could gimme an answer and explanation to both or either one of the following questions that'd be great.. thanx for any help guys:
Copper atoms crystallize in a unit cell of a cubic close packing lattice. How many copper atoms are contained in a single unit cell?
Europium crystalizes in a body-centered cubic system with atoms at all lattice points and an edge length of 4.58 angstroms. Calculate its density in g/cm3.
2 AnswersChemistry1 decade agoi've a few quick questions bout chemistry that i'm struggling with.. look below:?
1. Write the molecular equation for the combination of aqueous solutions of sodium chloride and silver nitrate.
2. Write the complete ionic equation for the combination of aqueous solutions of sodium chloride and silver nitrate.
3. Write the net ionic equation for the combination of aqueous solutions of sodium chloride and silver nitrate.
i know it looks like i'm doin my hw here but just need some help and some explanations.. any answers would be great thanx..
7 AnswersChemistry1 decade agochem question: if anyone can answer this and explain it that'd be great..?
Arrange the following bonds in order of increasing length (shortest first): (A) N---N, (B) Cl---N, (C) N-triple-bond-N, (D) C-triple-bond-N, and (E) H---N.
thanx for any answers guys..
3 AnswersChemistry1 decade ago